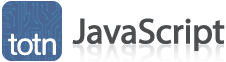
JavaScript: Variables
This JavaScript tutorial explains how to declare and use variables with syntax and examples.
Description
Variables are used to store data in your JavaScript code. Before you can use a variable in JavaScript, you must first declare it. Let's have a look at how to declare a variable.
Syntax
In JavaScript, the syntax for declaring a variable is:
var variable_name [= new_value];
Parameters or Arguments
- variable_name
- The name of the variable to declare. This is the identifier for the variable and it is case-sensitive. You should not use a reserved word as a variable name.
- new_value
- Optional. If provided, it is the value to assign to variable_name. If the value is a string, it must be enclosed in either single quotes (') or double quotes (").
Example
Let's take a look at some examples of how to declare a string, number and boolean variable in JavaScript.
Declare a String Variable
You can define a variable and assign a string value to it in a single declaration.
For example:
var h = 'TechOnTheNet';
or
var h = "TechOnTheNet";
The variable named h has been declared and given the string value of 'TechOnTheNet'. Notice that you can use either single quotes (') or double quotes (") when dealing with string values.
You do not have to declare and assign a value to the variable in one statement. Instead, you could first declare the variable named h, at which point, the h variable would have no value. Then you could assign a value to the h variable with a second statement as follows:
var h;
h = 'TechOnTheNet';
In this example, the var keyword declares the variable called h and the =
assigns the string value of "TechOnTheNet" to the h variable.
Declare a Number Variable
You can also define a variable and assign a number value to it in a single declaration.
For example:
var counter = 15;
In JavaScript, you can declare a variable named counter and give it the numeric value of 15.
As an alternative, you could use two statements to declare and assign the value. The first statement will declare the variable and the second statement will assign its value as follows:
var counter;
counter = 15;
Declare a Boolean Variable
You can also define a variable and assign a Boolean value to it in a single declaration.
For example:
var found = false;
In JavaScript, you can declare a variable named found and give it the Boolean value of false.
As an alternative, you could use two statements to declare and assign the value. The first statement will declare the variable and the second statement will assign its value as follows:
var found;
found = false;
Declare Multiple Variables
You can also define multiple variables and assign their values in a single declaration.
For example:
var h = 'TechOnTheNet', counter = 15, found = false;
In JavaScript, you can declare three variables named h, counter and found with the same var keyword and assign their values in a single statement.
This would be equivalent to the following 6 statements:
var h;
var counter;
var found;
h = 'TechOnTheNet';
counter = 15;
found = false;
Advertisements