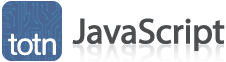
JavaScript: String includes() method
This JavaScript tutorial explains how to use the string method called includes() with syntax and examples.
Description
In JavaScript, includes() is a string method that determines whether a substring is found in a string. Because the includes() method is a method of the String object, it must be invoked through a particular instance of the String class.
Syntax
In JavaScript, the syntax for the includes() method is:
string.includes(substring [, start_position]);
Parameters or Arguments
- substring
- It is the substring that you want to search for within string.
- start_position
- Optional. It is the position in string where the search will start. The first position in string is 0. If this parameter is not provided, the search will start at the beginning of string.
Returns
The includes() method returns true if the substring is found in string. Otherwise, it returns false.
Note
- The includes() method performs a case-sensitive search.
- The includes() method does not change the value of the original string.
Example
Let's take a look at an example of how to use the includes() method in JavaScript.
For example:
var totn_string = 'TechOnTheNet';
console.log(totn_string.includes('e'));
In this example, we have declared a variable called totn_string that is assigned the string value of 'TechOnTheNet'. We have then invoked the includes() method of the totn_string variable to determine if a substring is found within totn_string.
We have written the output of the includes() method to the web browser console log, for demonstration purposes, to show what the includes() method returns.
The following will be output to the web browser console log:
true
In this example, the includes() method returned true because the substring 'e' is found within 'TechOnTheNet' when performing a case-sensitive search.
Specifying a Start Position Parameter
You can change the position where the search will start in the string by providing a start_position parameter to the includes() method.
For example:
var totn_string = 'TechOnTheNet';
console.log(totn_string.includes('e',11));
The following will be output to the web browser console log:
false
In this example, we have set the start_position parameter to a value of 11. This means that the search will begin looking for the value 'e' starting at position 11 in the string 'TechOnTheNet'. So in this case, the includes() method returned false because the substring 'e' is not found.
Specifying Multiple Characters as the Substring
Finally, the includes() method can search for multiple characters in a string.
For example:
var totn_string = 'TechOnTheNet';
console.log(totn_string.includes('The'));
The following will be output to the web browser console log:
true
In this example, the includes() method returned true because the substring 'The' is found in the string 'TechOnTheNet'.
Advertisements