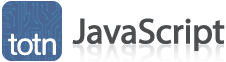
JavaScript: Number parseInt() method
This JavaScript tutorial explains how to use the Number method called parseInt() with syntax and examples.
Description
In JavaScript, parseInt() is a Number method that is used to parse a string and return its value as an integer number. Because parseInt() is a method of the Number object, it must be invoked through the object called Number.
Syntax
In JavaScript, the syntax for the parseInt() method is:
Number.parseInt(string_value [, radix]);
Parameters or Arguments
- string_value
- The string value to convert to an integer number.
- radix
Optional. It represents the radix or base of the mathematical numeral system to use to convert the string_value to an integer. It can be an integer value between 2 and 36.
For example, a radix of 2 would mean the string_value should be evaluated as a Binary number, a radix of 10 would mean that the string_value should be evaluated as a Decimal number, and so on.
If no radix is provided, the string_value will be evaluated as a Decimal number.
Returns
The parseInt() method parses a string and returns its value as an integer number (evaluating the string_value based on the mathematical numeral system specified by the optional radix parameter).
If the parseInt() method is passed a value that can not be converted to an integer using the appropriate radix (ie: Decimal is the default when no radix is provided), it will return NaN.
Note
- In mathematical numeral systems, the radix specifies the number of unique digitals in the numeral system including 0. For example, a radix of 10 would represent the Decimal system because the Decimal system uses ten digits from 0 through 9. Whereas, a radix of 2 would represent the Binary system because the Binary system uses only two digits which are 0 and 1.
Example
Let's take a look at an example of how to use the parseInt() method in JavaScript.
For example:
console.log(Number.parseInt('35.6'));
console.log(Number.parseInt('123ABC4'));
console.log(Number.parseInt('ABC123'));
In this example, we have invoked the parseInt() method using the Number class.
We have written the output of the parseInt() method to the web browser console log, for demonstration purposes, to show what the parseInt() method returns.
The following will be output to the web browser console log:
35 123 NaN
In this example, the first output to the console log returned 35 which is the integer representation of the string value '35.6'.
The second output to the console log returned 123 since the parseInt() method will parse the string value '123ABC4' until a non-numeric character is encountered and then it will discard the remainder of the string, returning the integer value.
The third output to the console log returned NaN since the string value 'ABC123' does not start with a numeric value.
Specifying a radix parameter
You can also specify a radix parameter to evaluate your string_value in a different mathematical numeral system than the Decimal system.
For example, let's evaluate our numbers as Binary:
console.log(Number.parseInt('111', 2));
console.log(Number.parseInt('24', 2));
The following will be output to the web browser console log:
7 NaN
In this example, we are using a radix of 2 to represent the Binary system. This means that our string_value parameter will be evaluated as a Binary number before calculating and returning its integer value.
The first output to the console log returned 7 which is the integer value of the Binary number 111 (represented as the string '111').
The second output to the console log returned NaN since the string value '24' does not represent a valid Binary number. A Binary nunber can only contain 0's and 1's.
Advertisements