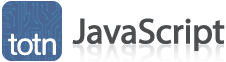
JavaScript: String split() method
This JavaScript tutorial explains how to use the string method called split() with syntax and examples.
Description
In JavaScript, split() is a string method that is used to split a string into an array of strings using a specified delimiter. Because the split() method is a method of the String object, it must be invoked through a particular instance of the String class.
Syntax
In JavaScript, the syntax for the split() method is:
string.split([delimiter [, count]]);
Parameters or Arguments
- delimiter
- Optional. It is delimiter used to break the string into the array of substrings. It can be a single character, string or regular expression. If this parameter is not provided, the split() method will return an array with one element containing the string.
- limit
- Optional. It determines the number of times the split will be performed using the delimiter. Once the limit has been reached, the split() method will discard the remainder of the string and it will not be returned as part of the array.
Returns
The split() method returns an array of strings that were split apart using the specified delimiter. The delimiter itself is not included in the resulting array elements.
Note
- The split() method does not change the value of the original string.
- If the delimiter is an empty string, the split() method will return an array of elements, one element for each character of string.
- If you specify an empty string for string, the split() method will return an empty string and not an array of strings.
Example
Let's take a look at an example of how to use the split() method in JavaScript.
For example:
var totn_string = 'Tech On The Net';
console.log(totn_string.split(' '));
console.log(totn_string.split(' ', 2));
In this example, we have declared a variable called totn_string that is assigned the string value of 'Tech On The Net'. We have then invoked the split() method of the totn_string variable to break the string into an array of strings using a delimiter.
We have written the output of the split() method to the web browser console log, for demonstration purposes, to show what the split() method returns.
The following will be output to the web browser console log:
["Tech", "On", "The", "Net"] ["Tech", "On"]
In the first call, we used a space character as the delimiter. In this example, the split() method returned an array of 4 elements consisting of the strings 'Tech', 'On', 'The', and 'Net'.
In the second call, we specified a limit of 2 as well as a space delimiter character. This time, the split() method returned an array of 2 elements consisting of 'Tech' and 'On' and discarded the remainder of the string after the limit of 2 splits was reached.
Using a Regular Expression as a Delimiter
Finally, we'll take a look at how to split a string into an array of strings using a regular expression.
For example:
var totn_string = 'Tech On The Net';
console.log(totn_string.split(/\s+/));
The following will be output to the web browser console log:
["Tech", "On", "The", "Net"]
In this example, we have used a regular expression for the delimiter so that we can split the string into words delimited by spaces. In this example, the split() method will return an array of 4 elements consisting of the strings 'Tech', 'On', 'The', and 'Net'.
Advertisements