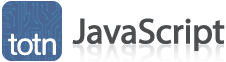
JavaScript: Number parseFloat() method
This JavaScript tutorial explains how to use the Number method called parseFloat() with syntax and examples.
Description
In JavaScript, parseFloat() is a Number method that is used to parse a string and return its value as a floating point number. Because parseFloat() is a method of the Number object, it must be invoked through the object called Number.
Syntax
In JavaScript, the syntax for the parseFloat() method is:
Number.parseFloat(string_value);
Parameters or Arguments
- string_value
- The string value to convert to a floating point number.
Returns
The parseFloat() method parses a string and returns its value as a floating point number.
If the parseFloat() method is passed a value that can not be converted to a floating point number, it will return NaN.
Example
Let's take a look at an example of how to use the parseFloat() method in JavaScript.
For example:
console.log(Number.parseFloat('25.678'));
console.log(Number.parseFloat('123ABC4'));
console.log(Number.parseFloat('ABC123'));
In this example, we have invoked the parseFloat() method using the Number class.
We have written the output of the parseFloat() method to the web browser console log, for demonstration purposes, to show what the parseFloat() method returns.
The following will be output to the web browser console log:
25.678 123 NaN
In this example, the first output to the console log returned 25.678 which is the floating point number representation of the string value '25.678'.
The second output to the console log returned 123 since the parseFloat() method will parse the string value '123ABC4' until a non-numeric character is encountered and then it will discard the remainder of the string.
The third output to the console log returned NaN since the string value 'ABC123' does not start with a numeric value.
Advertisements