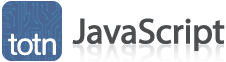
JavaScript: Number isInteger() method
This JavaScript tutorial explains how to use the Number method called isInteger() with syntax and examples.
Description
In JavaScript, isInteger() is a Number method that is used to return a Boolean value indicating whether a value is an integer. Because isInteger() is a method of the Number object, it must be invoked through the object called Number.
Syntax
In JavaScript, the syntax for the isInteger() method is:
Number.isInteger(value);
Parameters or Arguments
- value
- The value to test whether it is an integer.
Returns
The isInteger() method returns true if the value is an integer. Otherwise, it returns false.
If the isInteger() method is passed a non-numeric value, it will return false. This is because the isInteger() method does NOT convert the value to a number before testing whether it is an integer.
Note
- The isInteger() method does NOT convert the value parameter to a numeric value before determining whether the value is an integer.
Example
Let's take a look at an example of how to use the isInteger() method in JavaScript.
For example:
console.log(Number.isInteger(25));
console.log(Number.isInteger(6.7));
console.log(Number.isInteger('25'));
In this example, we have invoked the isInteger() method using the Number class.
We have written the output of the isInteger() method to the web browser console log, for demonstration purposes, to show what the isInteger() method returns.
The following will be output to the web browser console log:
true false false
In this example, the first output to the console log returned true since 25 is an integer.
The second output to the console log returned false since 6.7 is not an integer.
The third output to the console log returned false since the string value '25' is not a number and the isInteger() method does not convert the value to a number before testing whether it is an integer.
Advertisements