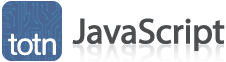
JavaScript: String charAt() method
This JavaScript tutorial explains how to use the string method called charAt() with syntax and examples.
Description
In JavaScript, charAt() is a string method that is used to retrieve a character at a specific position in a string. Because the charAt() method is a method of the String object, it must be invoked through a particular instance of the String class.
Syntax
In JavaScript, the syntax for the charAt() method is:
string.charAt([position]);
Parameters or Arguments
- position
- Optional. It is the position of the character in string that you wish to retrieve. The first position in the string is 0. If this parameter is not provided, the charAt() method will use 0 as the default.
Returns
The charAt() method returns a string representing a character at a specific position in a string.
The position must be between 0 and string.length-1. If the position is out of bounds, the charAt() method will return an empty string.
Note
- The charAt() method does not change the value of the original string.
Example
Let's take a look at an example of how to use the charAt() method in JavaScript.
For example:
var totn_string = 'TechOnTheNet';
console.log(totn_string.charAt(0));
console.log(totn_string.charAt(1));
console.log(totn_string.charAt(2));
console.log(totn_string.charAt(3));
In this example, we have declared a variable called totn_string that is assigned the string value of 'TechOnTheNet'. We have then invoked the charAt() method of the totn_string variable to return a character at a specific position.
We have written the output of the charAt() method to the web browser console log, for demonstration purposes, to show what the charAt() method returns.
The following will be output to the web browser console log:
T e c h
As you can see, the charAt() method returned a single character from the string in all four cases. The first call to the charAt() method returned "T" which is the character at position 0 of the string. The second call returned "e" which is the character at position 1. The third call returned "c" which is the character at position 2. The fourth call returned "h" which is the character at position 3.
No Parameter is Provided
Next, let's see what happens if you don't provide a position parameter to the charAt() method.
For example:
var totn_string = 'TechOnTheNet';
console.log(totn_string.charAt());
The following will be output to the web browser console log:
T
When no position parameter is provided, the charAt() method will use 0 as the value of the position parameter. In this example, the charAt() method returned the first character in the string (which is position 0) when no parameter was passed to the method.
Parameter is Out of Bounds
Finally, let's see what happens if the charAt() method is passed a position value that is out of bounds.
For example:
var totn_string = 'TechOnTheNet';
console.log(totn_string.charAt(85));
The following will be output to the web browser console log:
<empty string>
Because the first position in the string is 0, the position parameter must be a value between 0 and string.length-1. If the position parameter is out of bounds and does not fall in this range, the charAt() method will return an empty string.
Since 85 is a position that is out of bounds for the string 'TechOnTheNet', the charAt() method returned an empty string in the above example.
Advertisements