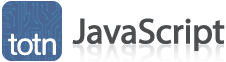
JavaScript: Number isSafeInteger() method
This JavaScript tutorial explains how to use the Number method called isSafeInteger() with syntax and examples.
Description
In JavaScript, isSafeInteger() is a Number method that is used to return a Boolean value indicating whether a value is a safe integer. This means that it is an integer value that can be exactly represented as an IEEE-754 double precision number without rounding. Because isSafeInteger() is a method of the Number object, it must be invoked through the object called Number.
Syntax
In JavaScript, the syntax for the isSafeInteger() method is:
Number.isSafeInteger(value);
Parameters or Arguments
- value
- The value to test whether it is a safe integer.
Returns
The isSafeInteger() method returns true if the value is a safe integer. Otherwise, it returns false.
If the isSafeInteger() method is passed a non-numeric value, it will return false. This is because the isSafeInteger() method does NOT convert the value to a number before testing whether it is an integer.
Note
- A safe integer is an integer value that can be exactly represented as an IEEE-754 double precision number without rounding. A safe integer can range in value from -9007199254740991 to 9007199254740991 (inclusive) which can be represented as
-(253 - 1)
to253 - 1
- The isSafeInteger() method does NOT convert the value parameter to a numeric value before determining whether the value is a safe integer.
Example
Let's take a look at an example of how to use the isSafeInteger() method in JavaScript.
For example:
console.log(Number.isSafeInteger(-9007199254740991));
console.log(Number.isSafeInteger(9007199254740991));
console.log(Number.isSafeInteger(-9007199254740992));
console.log(Number.isSafeInteger(9007199254740992));
In this example, we have invoked the isSafeInteger() method using the Number class.
We have written the output of the isSafeInteger() method to the web browser console log, for demonstration purposes, to show what the isSafeInteger() method returns.
The following will be output to the web browser console log:
true true false false
In this example, the first output to the console log returned true since -9007199254740991 is within the safe integer range of -9007199254740991 to 9007199254740991.
The second output to the console log also returned true since 9007199254740991 is within the safe integer range of -9007199254740991 to 9007199254740991.
The third and fourth output to the console log returned false since the values -9007199254740992 and 9007199254740992 are outside of the safe integer range of -9007199254740991 to 9007199254740991.
Advertisements