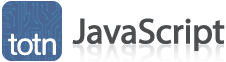
JavaScript: Math random() function
This JavaScript tutorial explains how to use the math function called random() with syntax and examples.
Description
In JavaScript, random() is a function that is used to return a pseudo-random number or random number within a range. Because the random() function is a static function of the Math object, it must be invoked through the placeholder object called Math.
Syntax
In JavaScript, the syntax for the random() function is:
Math.random();
Parameters or Arguments
There are no parameters or arguments for the random() function.
Returns
The random() function returns a value between 0 (inclusive) and 1 (exclusive), so value >= 0 and value < 1.
Note
- Math is a placeholder object that contains mathematical functions and constants of which random() is one of these functions.
Example
Let's take a look at an example of how to use the random() function in JavaScript.
For example:
console.log(Math.random());
In this example, we have invoked the random() function using the Math class.
We have written the output of the random() function to the web browser console log, for demonstration purposes, to show what the random() function returns.
The following will be output to the web browser console log:
0.0390260436146006
In this example, the first output to the console log returned 0.0390260436146006 which is a random number >= 0 and < 1.
(you will most likely see a different result from the random() function and not the value 0.0390260436146006).
Random Decimal Range
To create a random decimal number between two values (range), you can use the following formula:
Math.random()*(b-a)+a;
Where a is the smallest number and b is the largest number that you want to generate a random number for.
console.log(Math.random()*(25-10)+10);
The formula above would generate a random decimal number >= 10 and < 25. (Note: this formula will never return a value of 25 because the random function will never return 1.)
The following will be output to the web browser console log:
11.94632888346256
The value 11.94632888346256 is a decimal number between 10 (inclusive) and 25 (exclusive).
(Note: the result you get will be different because the random() function returns a random number)
Random Integer Range
To create a random integer number between two values (inclusive range), you can use the following formula:
Math.floor(Math.random()*(b-a+1))+a;
Where a is the smallest number and b is the largest number that you want to generate a random number for.
console.log(Math.floor(Math.random()*(25-10+1))+10);
The formula above would generate a random integer number between 10 and 25, inclusive.
The following will be output to the web browser console log:
15
The value 15 is an integer number between 10 (inclusive) and 25 (inclusive).
(Note: the result you get will be different because the random() function returns a random number)
Advertisements