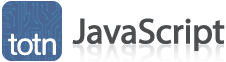
JavaScript: String lastIndexOf() method
This JavaScript tutorial explains how to use the string method called lastIndexOf() with syntax and examples.
Description
In JavaScript, lastIndexOf() is a string method that is used to find the location of a substring in a string, searching the string backwards. Because the lastIndexOf() method is a method of the String object, it must be invoked through a particular instance of the String class.
Syntax
In JavaScript, the syntax for the lastIndexOf() method is:
string.lastIndexOf(substring [, start_position]);
Parameters or Arguments
- substring
- It is the substring that you want to find within string.
- start_position
- Optional. It is the position in string where the search will start. The first position in string is 0 and the last position in string is string.length-1. If this parameter is not provided, the search for substring begins at the end of string and the full string is searched.
Returns
The lastIndexOf() method returns the position of the first occurrence of substring in string when searching the string backwards. The first position in the string is 0.
If the lastIndexOf() method does not find the substring in string, it will return -1.
Note
- The lastIndexOf() method performs a case-sensitive search.
- Even though the lastIndexOf() method searches the string backwards, it still returns a location value that is relative to the start of the string. For example, a return value of 0 is the location of the first character in the string, a return value of 1 is the location of the second character in the string, and so on.
- The lastIndexOf() method does not change the value of the original string.
Example
Let's take a look at an example of how to use the lastIndexOf() method in JavaScript.
For example:
var totn_string = 'TechOnTheNet';
console.log(totn_string.lastIndexOf('T'));
In this example, we have declared a variable called totn_string that is assigned the string value of 'TechOnTheNet'. We have then invoked the lastIndexOf() method of the totn_string variable to look for a substring within totn_string searching backwards.
We have written the output of the lastIndexOf() method to the web browser console log, for demonstration purposes, to show what the lastIndexOf() method returns.
The following will be output to the web browser console log:
6
In this example, the lastIndexOf() method returned 6. Because the string is search backwards, it found the first occurrence of T' within 'TechOnTheNet' in position 6 of the string.
Specifying a Start Position Parameter
You can change the position where the search will start in the string by providing a start_position parameter to the lastIndexOf() method.
For example:
var totn_string = 'TechOnTheNet';
console.log(totn_string.lastIndexOf('T',4));
The following will be output to the web browser console log:
0
In this example, we have set the start_position parameter to a value of 4. This means that the search will begin looking for the value 'T' starting at position 4 in the string and search the string backwards from there. So in this case, the substring 'T' is found at position 0 in the string 'TechOnTheNet'.
Specifying Multiple Characters as the Substring
Next, the lastIndexOf() method can search for multiple characters in a string.
For example:
var totn_string = 'TechOnTheNet';
console.log(totn_string.lastIndexOf('ch'));
The following will be output to the web browser console log:
2
In this example, the lastIndexOf() method returned 2 which is the position of 'ch' in the string 'TechOnTheNet' when searching the string in reverse (ie: from end to beginning of string).
Since the lastIndexOf() method can only return one value, it will return the position of the substring's first character when the occurrence is found, even though the substring is multiple characters in length.
No Occurrence is Found
Finally, the lastIndexOf() method will return -1 if an occurrence of substring is not found in string.
For example:
var totn_string = 'TechOnTheNet';
console.log(totn_string.lastIndexOf('z'));
The following will be output to the web browser console log:
-1
In this example, the lastIndexOf() method returned -1 because the substring 'z' is not found in the string 'TechOnTheNet'.
Advertisements