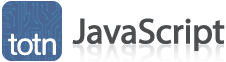
JavaScript: Array concat() method
This JavaScript tutorial explains how to use the Array method called concat() with syntax and examples.
Description
In JavaScript, concat() is an Array method that is used to concatenate two or more arrays into a new array. Because the concat() method is a method of the Array object, it must be invoked through a particular instance of the Array class.
Syntax
In JavaScript, the syntax for the concat() method is:
array.concat(value1, value2, ... value_n);
Parameters or Arguments
- value1, value2, ... value_n
- Optional. The values or arrays that will be concatenated with array.
Returns
The concat() method returns a new array with the elements of the calling array followed by the concatenation of each of the parameters, in their respective order.
Note
- The concat() method does not change the original array or any of the values provided as parameters.
Example
Let's take a look at an example of how to use the concat() method in JavaScript.
For example:
var totn_array1 = ['Tech','On'];
var totn_array2 = ['The','Net'];
console.log(totn_array1.concat(totn_array2));
In this example, we have declared two array objects called totn_array1 and totn_array2, each with 2 elements. We have then invoked the concat() method of the totn_array1 variable to return a new array that concatenates the two arrays together.
We have written the output of the concat() method to the web browser console log, for demonstration purposes, to show what the concat() method returns.
The following will be output to the web browser console log:
["Tech", "On", "The", "Net"]
In this example, the concat() method will return a new array of 4 elements consisting of the strings "Tech", "On", "The", and "Net".
Concatenating Values to the Array
The concat() method can also concatenate values to an array.
For example:
var totn_array = ['Tech','On','The','Net'];
console.log(totn_array.concat(1,2,'a','b'));
The following will be output to the web browser console log:
["Tech", "On", "The", "Net", 1, 2, "a", "b"]
In this example, we have used the concat() method to create a new array that concatenates the array ['Tech','On','The','Net'] with the values 1, 2, "a" and "b". This creates a new array with 8 elements. Notice that you can concatenation different types of elements - some of the elements in the new array are string values while others are numeric values.
Advertisements