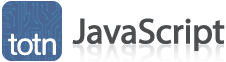
JavaScript: Array copyWithin() method
This JavaScript tutorial explains how to use the Array method called copyWithin() with syntax and examples.
Description
In JavaScript, copyWithin() is an Array method that is used to copy a portion of an array from one location in the array to another location in the same array. Because the copyWithin() method is a method of the Array object, it must be invoked through a particular instance of the Array class.
Syntax
In JavaScript, the syntax for the copyWithin() method is:
array.copyWithin(destination, copy_start, copy_end);
Parameters or Arguments
- destination
- The index location in the array to copy a portion of the array to. If destination is negative, the destination will be determined by applying the index location (in reverse) starting from the end of the array.
- copy_start
- Optional. The index position where the copy of the elements will begin. If copy_start is negative, the index position will be applied (in reverse) starting from the end of the array. If this parameter is not provided, it will default to 0.
- copy_end
- Optional. The index position where the copy of the elements will end, but does not including the ending element itself. If copy_end is negative, the index position will be applied (in reverse) starting from the end of the array. If this parameter is not provided, it will default to arr.length.
Returns
The copyWithin() method returns the modified array with the elements copied as specified by the destination, copy_start and copy_end parameters.
Note
- The copyWithin() method modifies the original array but it does not change the length of the array.
Example
Let's take a look at an example of how to use the copyWithin() method in JavaScript.
For example:
var totn_array = ['totn','a','b','c','d','e'];
console.log(totn_array.copyWithin(5, 0, 1));
In this example, we have declared an array object called totn_array that has 6 elements. We have then invoked the copyWithin() method of the totn_array variable to modify this array.
We have written the output of the copyWithin() method to the web browser console log, for demonstration purposes, to show what the copyWithin() method returns.
The following will be output to the web browser console log:
["totn", "a", "b", "c", "d", "totn"]
In this example, the copyWithin() method will return the modified array after copying the first element "totn" at index position 0 into the last element at index position 5.
Using Negative Parameter Values
When you use negative parameters, the copyWithin() method will determine the index positions starting from the end of the array.
For example:
var totn_array = ['a','b','c','d','e','totn'];
console.log(totn_array.copyWithin(-2, -1));
The following will be output to the web browser console log:
["a", "b", "c", "d", "totn", "totn"]
In this example, the copyWithin() method will return the modified array after copying the last element "totn" at index position -1 into the second last element at index position -2.
Advertisements