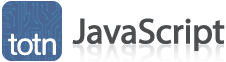
JavaScript: For-In Loop
This JavaScript tutorial explains how to use the for-in loop with syntax and examples.
Description
In JavaScript, the for-in loop is a basic control statement that allows you to loop through the properties of an object. The statements of code found within the loop body will be executed once for each property of the object.
Syntax
The syntax for the for-in loop in JavaScript is:
for (variable in object) {
// statements
}
Parameters or Arguments
- variable
- The name of a variable, an element of an array, or the property of an object.
- object
- A valid object or name of an object whose properties will be iterated through.
- statements
- The statements of code to execute each pass through the loop.
Note
- The body of the for-in loop will execute once for each property of the object.
- The for-in loop does not loop through all of the properties of the object. It is can only loop through the enumerable properties such as user-defined properties or inherited user-defined properties. Any properties that are flagged as non-enumerable (such as built-in properties or methods) will not be iterated through using the for-in loop.
- Since there is no specific order that the object properties are enumerated in the for-in loop, JavaScript may iterate through the properties in a different order depending on your implementation or version of JavaScript.
- See also the break statement to exit from the for-in loop early.
Example
Let's look at an example that shows how to use a for-in loop in JavaScript.
For example:
var totn_colors = { primary: 'blue', secondary: 'gray', tertiary: 'white' };
for (var color in totn_colors) {
console.log(totn_colors[color]);
}
In this example, the following will be output to the web browser console log:
blue gray white
Using the for-in loop with an Array
Since an array is an object, you can use the for-in loop to iterate through the elements of the array.
The following is the same example as above, but using an array:
var totn_colors = [ 'blue', 'gray', 'white' ];
for (var color in totn_colors) {
console.log(totn_colors[color]);
}
In this example, the following will be output to the web browser console log:
blue gray white
Advertisements