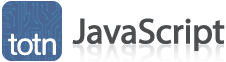
JavaScript: String fromCharCode() method
This JavaScript tutorial explains how to use the string method called fromCharCode() with syntax and examples.
Description
In JavaScript, fromCharCode() is a string method that is used to create a string from a sequence of Unicode values. Because the fromCharCode() method is a static method of the String constructor, it must be invoked through the String constructor object rather than through the particular instance of the String class.
Syntax
In JavaScript, the syntax for the fromCharCode() method is:
String.fromCharCode(value1, value2, ... value_n);
Parameters or Arguments
- value1, value2, ... value_n
- The Unicode values to convert to characters and then concatenate together into a string.
Returns
The fromCharCode() method accepts a sequence of Unicode values. These Unicode values are UTF-16 values (16-bit integers between 0 and 65535) that are converted to characters and concatenated together in a string. This resulting string is then returned by the fromCharCode() method.
Note
- The fromCharCode() method does not change the value of the original string.
- Use the fromCodePoint() method if any of the Unicode values you wish to convert are not representable in a single UTF-16 code unit.
Example
Let's take a look at an example of how to use the fromCharCode() method in JavaScript.
For example:
console.log(String.fromCharCode(84,101,99,104,79,110,84,104,101,78,101,116));
In this example, we have invoked the fromCharCode() static method through the String constructor object. This method will convert the 12 Unicode values to characters and then concatenate them into a single string result.
We have written the output of the fromCharCode() method to the web browser console log, for demonstration purposes, to show what the fromCharCode() method returns.
The following will be output to the web browser console log:
TechOnTheNet
As you can see, the fromCharCode() method converted the 12 Unicode values into their corresponding characters, concatenated them together into a single string, and then returned the resulting string.
84 = 'T'
101 = 'e'
99 = 'c'
104 = 'h'
79 = 'O'
110 = 'n'
84 = 'T'
104 = 'h'
101 = 'e'
78 = 'N'
101 = 'e'
116 = 't'
This makes the resulting string 'TechOnTheNet'.
Advertisements