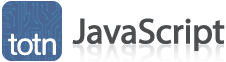
JavaScript: For Loop
This JavaScript tutorial explains how to use the for loop with syntax and examples.
Description
In JavaScript, the for loop is a basic control statement that allows you to execute code repeatedly for a fixed number of times.
Syntax
The syntax for the for loop in JavaScript is:
for (initialize; test; increment) {
// statements
}
Parameters or Arguments
- initialize
- The declaration of the counter variable and assigning its initial value. For example, to initialize a variable called counter and set its value to 1, you could use
var counter = 1;
- test
- The condition that is tested each pass through the loop. If the condition evaluates to TRUE, the loop body is executed. If the condition evaluates to FALSE, the loop is terminated. For example,
counter < 5;
- increment
- The increment value for the loop. You can increment or decrement the counter. For example, you could use
counter++
to increment the counter by 1 or you could usecounter--
to decrement the counter by 1. - statements
- The statements of code to execute each pass through the loop.
Note
- You would use a for loop when you want to execute the loop body a fixed number of times.
- See also the break statement to exit from the for loop early.
Example
Let's look at an example that shows how to use a for loop in JavaScript.
For example:
for (var counter = 1; counter < 5; counter++) {
console.log(counter + ' - Inside for loop on TechOnTheNet.com');
}
console.log(counter + ' - Done for loop on TechOnTheNet.com');
In this for loop example, the loop will continue as long as counter is less than 5 as specified by:
counter < 5
;
The for loop will continue while counter < 5. And once counter is >= 5, the loop will terminate.
In this example, the following will be output to the web browser console log:
1 - Inside for loop on TechOnTheNet.com 2 - Inside for loop on TechOnTheNet.com 3 - Inside for loop on TechOnTheNet.com 4 - Inside for loop on TechOnTheNet.com 5 - Done for loop on TechOnTheNet.com
Declare the counter variable outside of the for loop
You are not required to declare your counter variable within the for loop. Instead, you could rewrite your code as follows:
var counter;
for (counter = 1; counter < 5; counter++) {
console.log(counter + ' - Inside for loop on TechOnTheNet.com');
}
console.log(counter + ' - Done for loop on TechOnTheNet.com');
In this for loop example, the counter variable is declared before the for loop statement as specified by:
var counter;
Decrement the counter variable in the for loop
Traditionally, most for loops have an incrementing counter, but you can also decrement the counter. Let's look at an example:
for (var counter = 5; counter > 0; counter--) {
console.log(counter + ' - Inside for loop on TechOnTheNet.com');
}
console.log(counter + ' - Done for loop on TechOnTheNet.com');
In this for loop example, the counter variable is decremented as specified by:
counter--
In this example, the following will be output to the web browser console log:
5 - Inside for loop on TechOnTheNet.com 4 - Inside for loop on TechOnTheNet.com 3 - Inside for loop on TechOnTheNet.com 2 - Inside for loop on TechOnTheNet.com 1 - Inside for loop on TechOnTheNet.com 0 - Done for loop on TechOnTheNet.com
More complex incrementing in the for loop
Although most for loop are incremented by 1, you can choose an increment other than 1. Let's look at an example where we use an increment of 5 in the for loop:
for (var counter = 0; counter <= 20; counter = counter + 5) {
console.log(counter + ' - Inside for loop on TechOnTheNet.com');
}
console.log(counter + ' - Done for loop on TechOnTheNet.com');
In this for loop example, the counter variable will start at 0. The counter variable will then increment by 5 each pass through the loop, as specified by:
counter = counter + 5
When the counter variable is greater than 20, the for loop will terminate.
In this example, the following will be output to the web browser console log:
0 - Inside for loop on TechOnTheNet.com 5 - Inside for loop on TechOnTheNet.com 10 - Inside for loop on TechOnTheNet.com 15 - Inside for loop on TechOnTheNet.com 20 - Inside for loop on TechOnTheNet.com 25 - Done for loop on TechOnTheNet.com
Advertisements