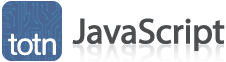
JavaScript: Array entries() method
This JavaScript tutorial explains how to use the Array method called entries() with syntax and examples.
Description
In JavaScript, entries() is an Array method that is used to return a new Array iterator object that allows you to iterate through the key/value pairs in the array. Because the entries() method is a method of the Array object, it must be invoked through a particular instance of the Array class.
Syntax
In JavaScript, the syntax for the entries() method is:
array.entries();
Parameters or Arguments
There are no parameters or arguments for the entries() method.
Returns
The entries() method returns a new Array iterator object that allows you to iterate through the key/value pairs in the array. The Array iterator object has a built-in method called next() that can be used to get the next value.
Example
Let's take a look at an example of how to use the entries() method in JavaScript.
For example:
var totn_array = ['Tech','On','The','Net'];
var totn_iterator = totn_array.entries();
console.log(totn_iterator.next().value);
console.log(totn_iterator.next().value);
console.log(totn_iterator.next().value);
console.log(totn_iterator.next().value);
In this example, we have declared an array object called totn_array that has 4 elements. We have then invoked the entries() method of the totn_array variable to create an Array iterator object called totn_iterator.
We have written the output of the next().value
methods of the Array iterator to the web browser console log, for demonstration purposes, to show what these calls return.
The following will be output to the web browser console log:
[0, "Tech"] [1, "On"] [2, "The"] [3, "Net"]
In this example, each of the calls will return the next entry in the Array iterator object. And if we called the next().value
method one more time (where there are no more entries), JavaScript would return a value of undefined.
undefined
Using a FOR Loop
Instead of using the next() method of the Array iterator object, you can also a for loop to iterator through the elements of the array.
For example:
var totn_array = ['Tech','On','The','Net'];
for (const [index, element] of totn_array.entries())
console.log(element);
The following will be output to the web browser console log:
"Tech" "On" "The" "Net"
In this example, the for loop will output each element in the array. We use the const
keyword to ensure that the elements of the array are not modified while looping through the for loop.
If you wanted to potentially change the array elements while looping, you would remove the const
keyword.
For example:
var totn_array = ['Tech','On','The','Net'];
for ([index, element] of totn_array.entries())
{
if (index == 0) element = "totn";
console.log(element);
}
The following will be output to the web browser console log:
"totn" "On" "The" "Net"
In this example, we are setting the first element in the array (where index is equal to 0) to "totn" while we are iterating through the elements. Therefore, we have omitted the const
keyword in the for loop. Otherwise, we would have raised an "Uncaught TypeError: Assignment to constant variable" error.
Advertisements