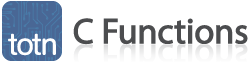
C Language: calloc function
(Allocate and Clear Memory Block)
In the C Programming Language, the calloc function allocates a block of memory for an array.
Syntax
The syntax for the calloc function in the C Language is:
void *calloc(size_t num_members, size_t size);
Parameters or Arguments
- num_members
- The number of elements in the array.
- size
- The size of the elements in bytes.
Note
- The block of memory is cleared by setting all bits to zero.
Returns
The calloc function returns a pointer to the beginning of the block of memory. If the block of memory can not be allocated, the calloc function will return a null pointer.
Required Header
In the C Language, the required header for the calloc function is:
#include <stdlib.h>
Applies To
In the C Language, the calloc function can be used in the following versions:
- ANSI/ISO 9899-1990
calloc Example
Let's look at an example to see how you would use the calloc function in a C program:
/* Example using calloc by TechOnTheNet.com */ /* The size of memory allocated MUST be larger than the data you will put in it */ #include <stdio.h> #include <stdlib.h> #include <string.h> int main(int argc, const char * argv[]) { /* Define required variables */ char *ptr; size_t length; /* Define the amount of memory required */ length = 50; /* Allocate and zero memory for our string */ ptr = (char *)calloc(length, sizeof(char)); /* Check to see if we were successful */ if (ptr == NULL) { /* We were not so display a message */ printf("Could not allocate required memory\n"); /* And exit */ exit(1); } /* Copy a string into the allocated memory */ strcpy(ptr, "C calloc at TechOnTheNet.com"); /* Display the contents of memory */ printf("%s\n", ptr); /* Free the memory we allocated */ free(ptr); return 0; }
When compiled and run, this application will output:
C calloc at TechOnTheNet.com
Similar Functions
Other C functions that are similar to the calloc function:
Advertisements