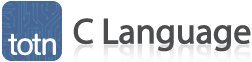
C Language: #include Directive
This C tutorial explains how to use the #include preprocessor directive in the C language.
Description
In the C Programming Language, the #include directive tells the preprocessor to insert the contents of another file into the source code at the point where the #include directive is found. Include directives are typically used to include the C header files for C functions that are held outsite of the current source file.
Syntax
The syntax for the #include directive in the C language is:
#include <header_file>
OR
#include "header_file"
- header_file
- The name of the header file that you wish to include. A header file is a C file that typically ends in ".h" and contains declarations and macro definitions which can be shared between several source files.
Note
- The difference between these two syntaxes is subtle but important. If a header file is included within <>, the preprocessor will search a predetermined directory path to locate the header file. If the header file is enclosed in "", the preprocessor will look for the header file in the same directory as the source file.
Example
Let's look at an example of how to use #include directives in your C program.
In the following example, we are using the #include directive to include the stdio.h header file which is required to use the printf standard C library function in your application.
/* Example using #include directive by TechOnTheNet.com */ #include <stdio.h> int main() { /* * Output "TechOnTheNet began in 2003" using the C standard library printf function * defined in the stdio.h header file */ printf("TechOnTheNet began in %d\n", 2003); return 0; }
In this example, the program will output the following:
TechOnTheNet began in 2003
Header Files
This is a list of the header files that you can include in your program if you want to use the functions available in the C standard library:
Header File | Type of Functions |
---|---|
<assert.h> | Diagnostics Functions |
<ctype.h> | Character Handling Functions |
<locale.h> | Localization Functions |
<math.h> | Mathematics Functions |
<setjmp.h> | Nonlocal Jump Functions |
<signal.h> | Signal Handling Functions |
<stdarg.h> | Variable Argument List Functions |
<stdio.h> | Input/Output Functions |
<stdlib.h> | General Utility Functions |
<string.h> | String Functions |
<time.h> | Date and Time Functions |
You will find these header files very useful as you become more familiar with the C language.
Advertisements