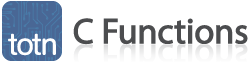
C Language: islower function
(Test for Lowercase Letter)
In the C Programming Language, the islower function tests whether c is a lowercase letter.
Syntax
The syntax for the islower function in the C Language is:
int islower(int c);
Parameters or Arguments
- c
- The value to test whether it is a lowercase letter.
Returns
The islower function returns a nonzero value if c is a lowercase letter and returns zero if c is not a lowercase letter.
Required Header
In the C Language, the required header for the islower function is:
#include <ctype.h>
Applies To
In the C Language, the islower function can be used in the following versions:
- ANSI/ISO 9899-1990
islower Example
Let's look at an example to see how you would use the islower function in a C program:
/* Example using islower by TechOnTheNet.com */ #include <stdio.h> #include <ctype.h> int main(int argc, const char * argv[]) { /* Define a variable to hold our test letter */ int letter; /* Set the letter to a lowercase 'b' */ letter = 'b'; /* Test to see if the letter provided is lowercase */ if (islower(letter)) printf("'%c' is lowercase\n", letter); else printf("'%c' is uppercase\n", letter); /* Set the letter to a uppercase 'B' */ letter = 'B'; /* Test to see if the letter provided is lowercase */ if (islower(letter)) printf("'%c' is lowercase\n", letter); else printf("'%c' is uppercase\n", letter); return 0; }
When compiled and run, this application will output:
'b' is lowercase 'B' is uppercase
Similar Functions
Other C functions that are similar to the islower function:
- isalpha function <ctype.h>
- isupper function <ctype.h>
- tolower function <ctype.h>
- toupper function <ctype.h>
See Also
Other C functions that are noteworthy when dealing with the islower function:
Advertisements