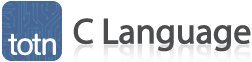
C Language: #error Directive
This C tutorial explains how to use the #error preprocessor directive in the C language.
Description
In the C Programming Language, the #error directive causes preprocessing to stop at the location where the directive is encountered. Information following the #error directive is output as a message prior to stopping preprocessing.
Syntax
The syntax for the #error directive in the C language is:
#error message
- message
- Message to output prior to stopping preprocessing.
Example
Let's look at how to use #error directives in your C program.
The following example shows the output of the #error directive:
/* Example using #error directive by TechOnTheNet.com */ #include <stdio.h> #include <limits.h> /* * Calculate the number of milliseconds for the provided age in years * Milliseconds = age in years * 365 days/year * 24 hours/day, 60 minutes/hour, 60 seconds/min, 1000 millisconds/sec */ #define MILLISECONDS(age) (age * 365 * 24 * 60 * 60 * 1000) int main() { /* The age of TechOnTheNet in milliseconds */ int age; #if INT_MAX < MILLISECONDS(12) #error Integer size cannot hold our age in milliseconds #endif /* Calculate the number of milliseconds in 12 years */ age = MILLISECONDS(12); printf("TechOnTheNet is %d milliseconds old\n", age); return 0; }
In this example, we are using the int data type to hold the age of TechOnTheNet in milliseconds. The int data type has a maximum value of INT_MAX which is defined in the limits.h header file and holds a value of 2^31 - 1 on both 32 and 64 bit systems. (See here for other variable types and their sizes.)
The statement #if INT_MAX < MILLISECONDS(12) evaluates whether or not the int data type is large enough to store the age in milliseconds. If it is not, the processor will output the following error message:
error: Integer size cannot hold our age in milliseconds
Since an error occurred, the program compilation will not complete and therefore no executable program will be created.
A typical use of the #error directive is to prevent compilation if a known condition exists that would cause the program to not function properly if the compilation completed. (For example, the C source was designed for a specific platform and was being compiled on an incompatible platform.)
Advertisements