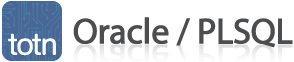
Oracle / PLSQL: EXISTS Condition
This Oracle tutorial explains how to use the Oracle EXISTS condition with syntax and examples.
The Oracle EXISTS condition is used in combination with a subquery and is considered "to be met" if the subquery returns at least one row. It can be used in a SELECT, INSERT, UPDATE, or DELETE statement.
Syntax
The syntax for the EXISTS condition in Oracle/PLSQL is:
WHERE EXISTS ( subquery );
Parameters or Arguments
- subquery
- The subquery is a SELECT statement. If the subquery returns at least one record in its result set, the EXISTS clause will evaluate to true and the EXISTS condition will be met. If the subquery does not return any records, the EXISTS clause will evaluate to false and the EXISTS condition will not be met.
Note
- Oracle SQL statements that use the Oracle EXISTS condition are very inefficient since the sub-query is RE-RUN for EVERY row in the outer query's table. There are more efficient ways to write most queries, that do not use the EXISTS condition.
Example - With SELECT Statement
Let's look at a simple example.
The following is a SELECT statement that uses the EXISTS condition:
SELECT * FROM customers WHERE EXISTS (SELECT * FROM order_details WHERE customers.customer_id = order_details.customer_id);
This Oracle EXISTS condition example will return all records from the customers table where there is at least one record in the order_details table with the matching customer_id.
Example - With SELECT Statement using NOT EXISTS
The Oracle EXISTS condition can also be combined with the NOT operator.
For example,
SELECT * FROM customers WHERE NOT EXISTS (SELECT * FROM order_details WHERE customers.customer_id = order_details.customer_id);
This Oracle EXISTS example will return all records from the customers table where there are no records in the order_details table for the given customer_id.
Example - With INSERT Statement
The following is an example of an INSERT statement that uses the EXISTS condition:
INSERT INTO contacts (contact_id, contact_name) SELECT supplier_id, supplier_name FROM suppliers WHERE EXISTS (SELECT * FROM order_details WHERE suppliers.supplier_id = order_details.supplier_id);
Example - With UPDATE Statement
The following is an example of an UPDATE statement that uses the EXISTS condition:
UPDATE suppliers SET supplier_name = (SELECT customers.name FROM customers WHERE customers.customer_id = suppliers.supplier_id) WHERE EXISTS (SELECT customers.name FROM customers WHERE customers.customer_id = suppliers.supplier_id);
Example - With DELETE Statement
The following is an example of a DELETE statement that uses the EXISTS condition:
DELETE FROM suppliers WHERE EXISTS (SELECT * FROM order_details WHERE suppliers.supplier_id = order_details.supplier_id);
Advertisements