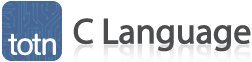
C Language: Variables
This C tutorial explains how to declare variables in the C language.
Description
In the C Programming Language, variables are used to store various types of data. Variables must be defined before they can be used within a program. Variable declarations include the type of the variable and, optionally, its initial value.
Syntax
The syntax for declaring a variable in the C language is:
c_type variable_name1 [= value1];
Additional variables of the same C type can be declared by comma separating the variables as follows:
c_type variable_name1 [= value1] [, variable_name2 [= value2], ... variable_name_n [= value_n]];
Parameters or Arguments
- c_type
- The type of the variable being declared. Refer to the Types section below for a list of the common C types.
- variable_name1
- The name of the first variable to declare.
- value1
- Optional. If provided, it is the value to assign to variable_name1.
- variable_name2, ... variable_name_n
- Optional. These are additional variables that will be declared with the same C type.
- value2, ... value_n
- Optional. These are the values that you wish to assign to variable_name2 through variable_name_n.
Note
- Declaration statements must end with a semicolon.
Constant Variable
To define a constant variable, you can place the keyword const in front of the declaration as follows:
const c_type variable_name1 [= value1];
Variables defined as constants can not be changed after their initial value is set.
Types
The following is a table of the most common variable types used in C. The min and max values are based on a 32-bit system:
C Type | Variable Type | Typically Holds | Min Value | Max Value |
---|---|---|---|---|
signed char | Signed Character | 7 bit ASCII character | -128 | 127 |
unsigned char | Unsigned Character | 8 bit ASCII character | 0 | 255 |
short int | Short Integer | Signed 16 bit Integer number | -32,768 | 32,767 |
unsigned short int | Unsigned Short Integer | Unsigned 16 bit Integer number | 0 | 65,535 |
int | Integer | Signed 32 bit Integer number | -2,147,483,648 | 2,147,483,647 |
unsigned int | Unsigned Integer | Unsigned 32 bit Integer number | 0 | 4,294,967,295 |
long int | Signed Long Integer | Signed 64 bit Integer number | -2,147,483,648 | 2,147,483,647 |
unsigned long int | Unsigned Long Integer | Unsigned 64 bit Integer number | 0 | 4,294,967,295 |
float | Float | Single precision floating point number | 1.17 x 10-38 | 3.4 x 1038 |
double | Double | Double precision floating point number | 2.2 x 10-308 | 1.79 x 10308 |
Let's take a closer look at how to define and use each of these C variable types.
Advertisements