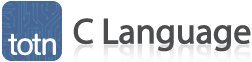
C Language: #elif Directive
This C tutorial explains how to use the #elif preprocessor directive in the C language.
Description
In the C Programming Language, the #elif provides an alternate action when used with the #if, #ifdef, or #ifndef directives. The preprocessor will include the C source code that follows the #elif statement when the condition of the preceding #if, #ifdef or #ifndef directive evaluates to false and the #elif condition evaluates to true.
The #elif directive can be thought of as #else if.
Syntax
The syntax for the #elif directive in the C language is:
#elif conditional_expression
- conditional_expression
- Expression that must evaluate to true for the preprocessor to include the C source code into the compiled application.
Note
- The #elif directive must be closed by an #endif directive.
Example
The following example shows how to use the #elif directive in the C language:
/* Example using #elif directive by TechOnTheNet.com */ #include <stdio.h> #define YEARS_OLD 12 int main() { #if YEARS_OLD <= 10 printf("TechOnTheNet is a great resource.\n"); #elif YEARS_OLD > 10 printf("TechOnTheNet is over %d years old.\n", YEARS_OLD); #endif return 0; }
In this example, YEARS_OLD has a value of 12 so the statement #if YEARS_OLD <=10 evaluates to false. As a result, processing is passed to the #elif YEARS_OLD > 10 statement which evaluates to true. The C source code following the #elif statement is then compiled into the application.
Here is the output of the executable program:
TechOnTheNet is over 12 years old.
Advertisements