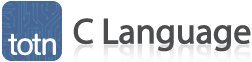
C Language: #ifndef Directive
This C tutorial explains how to use the #ifndef preprocessor directive in the C language.
Description
In the C Programming Language, the #ifndef directive allows for conditional compilation. The preprocessor determines if the provided macro does not exist before including the subsequent code in the compilation process.
Syntax
The syntax for the #ifndef directive in the C language is:
#ifndef macro_definition
- macro_definition
- The macro definition that must not be defined for the preprocessor to include the C source code into the compiled application.
Note
- The #ifndef directive must be closed by an #endif directive.
Example
The following example shows how to use the #ifndef directive in the C language:
/* Example using #ifndef directive by TechOnTheNet.com */ #include <stdio.h> #define YEARS_OLD 12 #ifndef YEARS_OLD #define YEARS_OLD 10 #endif int main() { printf("TechOnTheNet is over %d years old.\n", YEARS_OLD); return 0; }
In this example, if the macro YEARS_OLD is not defined before the #ifndef directive is encountered, it will be defined with a value of 10.
Here is the output of the executable program:
TechOnTheNet is over 12 years old.
If you remove the line #define YEARS_OLD 12, you will see the following output from the executable program:
TechOnTheNet is over 10 years old.
Advertisements