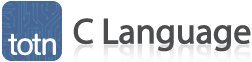
C Language: #undef Directive
This C tutorial explains how to use the #undef preprocessor directive in the C language.
Description
In the C Programming Language, the #undef directive tells the preprocessor to remove all definitions for the specified macro. A macro can be redefined after it has been removed by the #undef directive.
Once a macro is undefined, an #ifdef directive on that macro will evaluate to false.
Syntax
The syntax for the #undef directive in the C language is:
#undef macro_definition
- macro_definition
- The name of the macro which will be removed by the preprocessor.
Example
The following example shows how to use the #undef directive:
/* Example using #undef directive by TechOnTheNet.com */ #include <stdio.h> #define YEARS_OLD 12 #undef YEARS_OLD int main() { #ifdef YEARS_OLD printf("TechOnTheNet is over %d years old.\n", YEARS_OLD); #endif printf("TechOnTheNet is a great resource.\n"); return 0; }
In this example, the YEARS_OLD macro is first defined with a value of 12 and then undefined using the #undef directive. Since the macro no longer exists, the statement #ifdef YEARS_OLD evaluates to false. This causes the subsequent printf function to be skipped.
Here is the output of the executable program:
TechOnTheNet is a great resource.
Advertisements