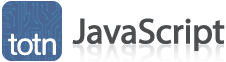
JavaScript: Number toString() method
This JavaScript tutorial explains how to use the Number method called toString() with syntax and examples.
Description
In JavaScript, toString() is a Number method that is used to convert a number to a string representation of the number. Because toString() is a method of the Number object, it must be invoked through a particular instance of the Number class.
Syntax
In JavaScript, the syntax for the toString() method is:
number.toString([radix]);
Parameters or Arguments
- radix
-
Optional. It represents the radix or base of the mathematical numeral system to use to convert the number to a string representation of the number. It can be an integer value between 2 and 36.
For example, a radix of 2 would mean the number should be returned as a string representation of a Binary number, a radix of 10 would mean that the number should be returned as a string representation of a Decimal number, and so on.
If no radix is provided, the number will be returned as a string representation of a Decimal number.
Returns
The toString() method returns a string representation of a number (in the mathematical numeral system specified by the optional radix parameter).
Note
- In mathematical numeral systems, the radix specifies the number of unique digitals in the numeral system including 0. For example, a radix of 10 would represent the Decimal system because the Decimal system uses ten digits from 0 through 9. Whereas, a radix of 2 would represent the Binary system because the Binary system uses only two digits which are 0 and 1.
- The toString() method does not change the value of the original number.
Example
Let's take a look at an example of how to use the toString() method in JavaScript.
For example:
var totn_number = 123.4;
console.log(totn_number.toString());
In this example, we have declared a variable called totn_number that is assigned the value of 123.4. We have then invoked the toString() method of the totn_number to convert the number to a string representation of the number.
We have written the output of the toString() method to the web browser console log, for demonstration purposes, to show what the toString() method returns.
The following will be output to the web browser console log:
123.4
In this example, the output to the console log returned "123.4" which is the string representation of the number 123.4. Since there is no radix parameter provided, it displays the Decimal representation of the number.
Specifying a radix parameter
You can also specify a radix parameter to display the result in a different mathematical numeral system than the Decimal system.
For example, let's return our result as a Binary number:
var totn_number = 13;
console.log(totn_number.toString(2));
console.log(totn_number.toString(8));
console.log(totn_number.toString(16));
The following will be output to the web browser console log:
1101 15 d
In this example, the first output to the console log returned "1101". Since we are using a radix of 2 to represent the Binary system, the number 13 will be converted to the Binary value of 1101 and returned as the string value "1101".
The second output to the console log returned "15". A radix of 8 represents the Octal system, so the number 13 will be converted to Octal and returned as the string value "15".
The third output to the console log returned "d". A radix of 16 represents the Hexadecimal system, so the number 13 will be converted to Hexadecimal and returned as the string value "d".
Advertisements